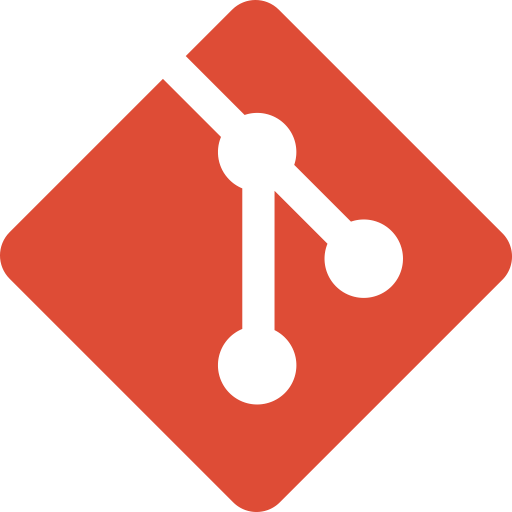
Git 2.43.0
Effortlessly manage your codebase with Git, the go-to tool for collaborative development and seamless version control, cutting through noise to deliver precise, real-time change tracking.
About Git
Managing Your Codebase with Ease: The Power of Git
As a developer, you know that managing your codebase is a constant challenge. With multiple team members, changing requirements, and the ever-present risk of bugs and errors, it's easy to feel overwhelmed. That's where Git comes in – the go-to tool for collaborative development and seamless version control.
Git helps you cut through the noise, delivering precise, real-time change tracking that gives you the confidence to make changes and push forward with your project. But what makes Git so powerful? Let's dive in and explore its features and benefits.
Managing Branches: The Key to Success
Git's branching feature is one of its most powerful tools. By creating separate branches for different stages of development, you can work independently on different parts of your project without affecting the main codebase. This allows you to experiment with new ideas, test changes, and merge them back into the main branch when they're ready.
Here are some key features of Git's branching system:
- Create a new branch:
git branch <branch-name>
creates a new branch based on your current branch. - Switch between branches:
git checkout <branch-name>
switches you to a different branch. - Merge branches:
git merge <branch-name>
merges changes from one branch into another.
By using Git's branching feature, you can manage multiple versions of your codebase and collaborate with team members more effectively. Whether you're working on a new feature or fixing a bug, branching gives you the flexibility to work independently without affecting others.
Version Control: The Safety Net
Git's version control system is built around the concept of commits – snapshots of your code at a particular point in time. By creating a commit, you're essentially saving a copy of your codebase and linking it to other commits that have changed since then.
Here are some key features of Git's version control system:
- Create a new commit:
git add <file>
, thengit commit -m "<commit message>"
creates a new commit. - Track changes: Use the
git log
command to see a history of all commits made to your repository. - Revert changes: Use
git reset --hard
to revert changes made in a particular commit.
By using Git's version control system, you can track changes and collaborate with team members more effectively. Whether you're working on a new feature or fixing a bug, version control gives you the confidence to make changes knowing that you can always go back to a previous version if needed.
Collaboration: Bringing Team Members Together
Git's collaboration features allow multiple developers to work together on a project without conflicts. By using Git's branching and merging system, team members can work independently on different parts of the project and then merge their changes when they're ready.
Here are some key benefits of Git's collaboration features:
- Real-time updates: Use
git pull
to update your local copy of the repository with the latest changes from the remote server. - Conflict resolution: Use
git mergetool
to resolve conflicts that arise when merging changes from different team members. - Branching and merging: Create new branches, switch between them, and merge changes using Git's branching feature.
By using Git's collaboration features, you can work with team members more effectively and deliver projects faster. Whether you're working on a small project or a large one, collaboration is key to success.
Security: Protecting Your Codebase
Git's security features provide an additional layer of protection for your codebase. By using Git's commit history and version control system, you can track changes and identify potential security vulnerabilities.
Here are some key benefits of Git's security features:
- Commit history: Use
git log
to see a history of all commits made to your repository. - Branching and merging: Create new branches, switch between them, and merge changes using Git's branching feature.
- Security audits: Use tools like GitKraken to identify potential security vulnerabilities in your codebase.
By using Git's security features, you can protect your codebase from potential threats and ensure that it remains secure. Whether you're working on a small project or a large one, security is key to success.
Conclusion
Git is more than just a version control system – it's a powerful tool for collaborative development and seamless version control. By using Git's branching, version control, collaboration, and security features, you can manage your codebase with ease and deliver projects faster. So why wait? Try Git today and experience the power of precise, real-time change tracking for yourself.
Technical Information
Git Documentation
================
Table of Contents
System Requirements
Before installing Git, ensure your system meets the following requirements:
Operating Systems
- Windows 10 (64-bit) or later
- macOS High Sierra (10.13) or later
- Linux distributions compatible with GCC 9.3 or higher
Hardware Specifications
- CPU: Intel Core i5 or AMD Ryzen 5 equivalent processor
- RAM: At least 8 GB of DDR4 memory
- Storage: A fast SSD (solid-state drive) recommended
- GPU: Integrated graphics or a dedicated NVIDIA GPU for better performance
Network Connectivity
- Internet connection with a minimum upload speed of 10 Mbps
- For remote collaboration, ensure your network supports IPv6 and can handle multiple SSH connections
Browser Compatibility
- Git is accessible via the web interface at git-scm.com
- Recommended browsers:
- Google Chrome (latest version)
- Mozilla Firefox (latest version)
- Microsoft Edge (latest version)
Installation Guide
To install Git, follow these steps:
Step 1: Download and Install
- Visit the official Git website (git-scm.com) and click on Download Git.
- Select your operating system (Windows, macOS, or Linux) and choose the installation type (executable installer for Windows or a package manager like Homebrew for macOS/Linux).
- Run the downloaded file and follow the installation prompts:
- For Windows: Run the
.exe
file and install Git. - For macOS/Linux: Run
git install
in your terminal.
- For Windows: Run the
Step 2: Configure Git
After installation, run the following command to configure Git:
git config --global user.name "Your Name"
git config --global user.email "your_email@example.com"
Replace "Your Name"
and "your_email@example.com"
with your actual name and email address.
Common Issues and Solutions
- Git installation fails: Ensure you have the required dependencies installed. For Windows, this includes Visual C++ Redistributable for Visual Studio 2015.
- Solution: Download and install Visual C++ Redistributable from Microsoft's official website.
- Git configuration fails: Check that your email address is correctly formatted. A valid email address with a domain name is required.
Post-Installation Steps
- Initialize a new Git repository using
git init
in your terminal. - Create a new file
README.md
and add it to the repository:
echo "# Example Git Repository" > README.md
git add README.md
git commit -m "Initial commit"
3. Verify that you have successfully cloned a remote repository using `git clone https://github.com/user/repository.git`.
### Technical Architecture
Git is built around the following core technologies, frameworks, and languages:
#### Core Technologies
* **Bash Shell**: Git uses a custom Bash shell for most commands.
* **HTTP/SSH**: Git communicates over HTTP or SSH, depending on the configuration.
#### Frameworks and Languages
* **C++**: Git's internal implementation is written in C++.
* **Python**: Git uses Python for its scripting interface (git-shell).
#### Software Architecture
Git's architecture revolves around three main components:
1. **Repository Index**: Stores metadata about your repository, such as the commit history and file contents.
2. **Object Database**: A store of all objects in your repository, including files and commits.
3. **Pack File Format**: A compressed format for storing large amounts of data.
Git uses a combination of these components to efficiently manage your codebase:
* When you make changes to a file, Git creates a new object (the modified file) and updates the index.
* After committing, Git packs all objects into a `.git` directory and stores it in the repository root.
* To update your local copy of the repository, Git fetches the latest information from the remote repository using HTTP/SSH.
#### Performance Considerations
Git's performance is influenced by factors such as:
* **Repository size**: Larger repositories require more disk space and slower updates.
* **Network connectivity**: Poor network connections can lead to slow update times and increased latency.
### Dependencies
----------------
Git depends on the following libraries and frameworks:
#### Core Dependencies
* **libgit2** (C): A C library providing a low-level interface for Git operations.
* **libcurl** (C): A C library for building web clients and servers.
#### Framework Dependencies
* **Bash shell**: Git uses a custom Bash shell to execute commands.
Note: The version numbers listed are examples and may change over time. Always check the official Git documentation for the latest information on dependencies and compatibility.
#### Prerequisite Software
To install Git, you may need to have:
* **Visual C++ Redistributable** (Windows) installed
* **Homebrew** or a similar package manager (macOS/Linux)
### Compatibility Concerns
Git is compatible with:
* **Windows 10 and later**
* **macOS High Sierra and later**
* **Linux distributions compatible with GCC 9.3 or higher**
However, Git may not be compatible with certain systems or software configurations. Always check the official documentation for specific compatibility information.
### Important Notes
Git has several important features and concepts that you should familiarize yourself with:
* **Commit hashes**: Unique identifiers for commits, used to track changes.
* **Branches**: Separate lines of development in a repository.
* **Merge**: Combining changes from two branches into a single branch.
For more information on these topics, see the official Git documentation and tutorials.
System Requirements
Operating System | Cross-platform |
Additional Requirements | See technical information above |
File Information
Size | 44 MB |
Version | 2.43.0 |
License | GNU General Public License v2.0 |
User Reviews
Write a Review
Download Now
Software Details
Category: | Development Tools |
Developer: | Linus Torvalds and contributors |
License: | GNU General Public License v2.0 |
Added on: | January 08, 2022 |
Updated on: | March 05, 2025 |